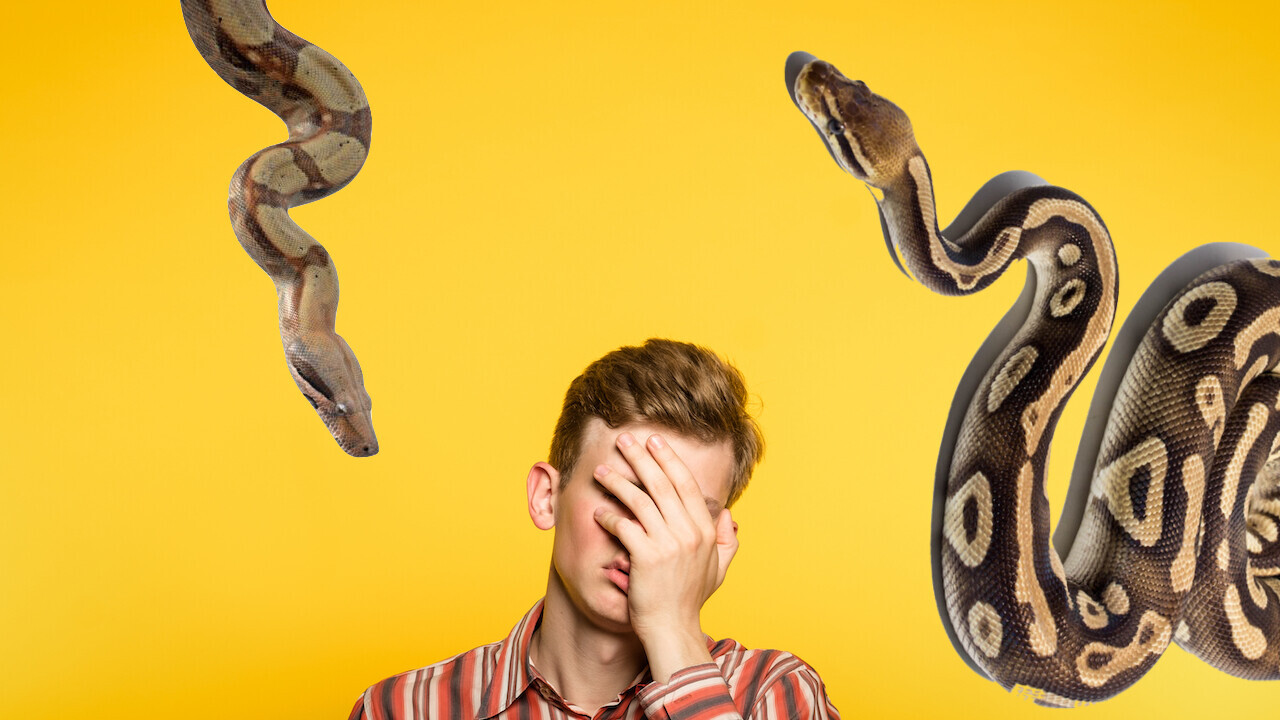
Fail fast, fail early — we’ve all heard the motto. Still, it’s frustrating when you’ve written a beautiful piece of code, just to realize that it doesn’t work as you’d expected.
That’s where unit tests come in. Checking each piece of your code helps you localize and fix your bugs.
But not all bugs are created the same. Some bugs are unexpected, not obvious to see at all, and hard to fix even for experienced developers. These are more likely to occur in large and complex projects, and spotting them early can save you a ton of time later on.
Other bugs are trivial, like when you’ve forgotten a closing bracket or messed up some indentations. They’re easy to fix, but hard to spot, especially when you’ve been working on the code for a while or when it’s late at night.
Once you’ve spotted a bug like this, it’s a bit of a facepalm moment. You could kick yourself for not having seen it earlier — and you wonder why you did such a stupid mistake in the first place. They’re also not the type of bug that you’d want your colleagues to spot before you do.
Silly but common mistakes to check for first
I can’t claim that this list covers all silly mistakes that you’ll ever make. However, using it regularly should at least help you eliminate the most common ones.
Forgot that closing brace?
It happens to everyone — you happily code away, and in the flow of it you forget to close that array, argument list, or whatever you’re dealing with.
Some developers type a closing brace as soon as they open one, and then fill the space in between. Most modern IDEs also close braces automatically — so if forgetting braces is a chronic disease of yours, you might consider leaving your old IDE behind.
What about your colons?
Hey, it happens to the best of us. You’re constructing a new class, and since it’s complex, you’re already thinking about the contents of the class while your typing the code. And whoops, you’ve forgotten that colon at the end:

A good rule of thumb is that if you’re increasing the indent of a line, you’ll need to add a colon to the line that comes before it.
Have you confused equality and assignment operators?
When do you use =
, and when do you use ==
? As a rule of thumb, if you’re checking or comparing two values, you’ll use ==
. On the other hand, you’ll use =
if you’re assigning a value to a variable.
Have you muddled with mutable expressions?
Have a look at this:
Python is case-sensitive, remember?
This is trivial as hell but happens all the time. Say you’ve defined the variable CamelBucket
, and later in the code you call camelbucket
.
Won’t work, huh? Chances are, you know very well that Python is case-sensitive, but you just forgot to press that shift key.
Most modern IDEs can help you avoid this mistake by making smart suggestions. So if you’re prone to typos, you might consider upgrading your text editor.
Are you modifying lists while iterating over them?
This has probably happened to every junior developer out there: you’ve built a list, and now you want to change a few things. No big deal, right?
Wrong. Consider this:

Spoiler alert: this throws an error because you end up iterating over items in a list that don’t exist anymore. Instead of deleting from an existing list, consider writing to a new one.
Do you have circular module dependencies?
This is so trivial, but also frustratingly common: in one file, let’s saybug.py
, you writeimport anotherbug
. In another file,anotherbug.py
, you writeimport bug
.
This isn’t going to work — how should the computer know which file to include in which? You probably knew this but did it by accident. No worries, but fix it asap!
You haven’t named your modules like Python’s standard libraries, right?
Another thing regarding modules: Python comes with a wealth of amazing library modules. But if you need to create your own, be sure to give them an original name.
For example, defining a module callednumpy.py
is going to lead to confusion. If you’re in doubt which names you can use, there’s a complete list of Python’s standard libraries.
How to avoid silly mistakes
You learn from your mistakes. As a beginner-level developer, you might still be making mistakes like these every day. But as time moves on, they become less and less.
You can also take it to the next level and adopt some healthy habits so you never make trivial mistakes. These are just a few tips of many, but they can help you avoid many bugs.
Always initialize variables
Python code works perfectly if you define a variable and don’t assign a value until later. It therefore seems cumbersome to initialize them every time upon definition.
But don’t forget that you’re human, and as such you’re prone to losing track of which variable has got assigned and which one hasn’t. Also, initializing variables forces you to think about what type of variable you’re dealing with, which might prevent bugs down the line.
That’s why many seasoned developers initialize every variable with default values such as 0
, None
, []
, ""
, and so on.
Of course, if you’re just trying to tie up a little standalone script and not working on a mammoth-sized project, you can allow yourself to do things quick and sloppy. But remember to be diligent when things get complex.
Use braces to call functions and avoid trouble
Braces again. Somehow they’re just not compatible with the sloppiness of human brains.
In Python, you’ll always need to call a function like this: callingthisfunction()
, and not like this: notcallingthisfunction
, whether there are any arguments or not. Sounds trivial, but isn’t always!
For example, if you have a file called file
and you want to close it in Python, you’ll write file.close()
. The code will run without throwing an error, though, if you write file.close
— except that it won’t close the file. Try to locate a mistake like that in a project with thousands of lines of code…
It becomes pretty automatic after a few days of practicing, so start today: functions are always called with braces!
Don’t use extensions while importing modules
Again, this is rather trivial but often forgotten by beginners. If you’re calling a module, you’ll never use an extension, regardless whether it’s from the Python standard library or elsewhere.
So watch out for lines like:
import numpy.py # don't do that!!!
Delete that .py
extension and avoid it like stink. Extensions and imports don’t work together.
Indent consistently
Again, you probably know yourself that you need to index the same way throughout a file. But it’s still a common pitfall when you’re collaborating with others or when it’s late at night.
Before you start coding, decide whether you use tabs or spaces, and how many spaces. The most common convention is four spaces, but you can do whatever suits you best — as long as you keep it up throughout the document.
If, on the other hand, you use spaces in one line and tabs in another, sometimes Python doesn’t treat that like you’d expected. I know it seems like a dumb job, but try to be as diligent as you can about that
The bottom line: avoid facepalm-moments for yourself and your colleagues
Fail fast, fail early — but don’t fail stupidly. Everybody makes mistakes, but still, it’s better to avoid the stupid ones, or at least fix them quickly.
It’s not only about making progress. It’s also about avoiding facepalm-moments where you kick yourself for not having spotted a bug earlier.
And it’s about not losing your reputation — who wants to run to a colleague or a manager with a seemingly complicated problem, only to find out that it was really easy to fix?
Chances are that you’ve done some of the mistakes I’ve mentioned in the past. Chill, buddy, I have, too — we’re human.
The point is, if your code behaves in unexpected ways, you’ll want to check for the trivial things first. Then, if your code still doesn’t work properly, you can always ping your colleague.
This article was written by Ari Joury and was originally published on Towards Data Science. You can read it here.
Get the TNW newsletter
Get the most important tech news in your inbox each week.